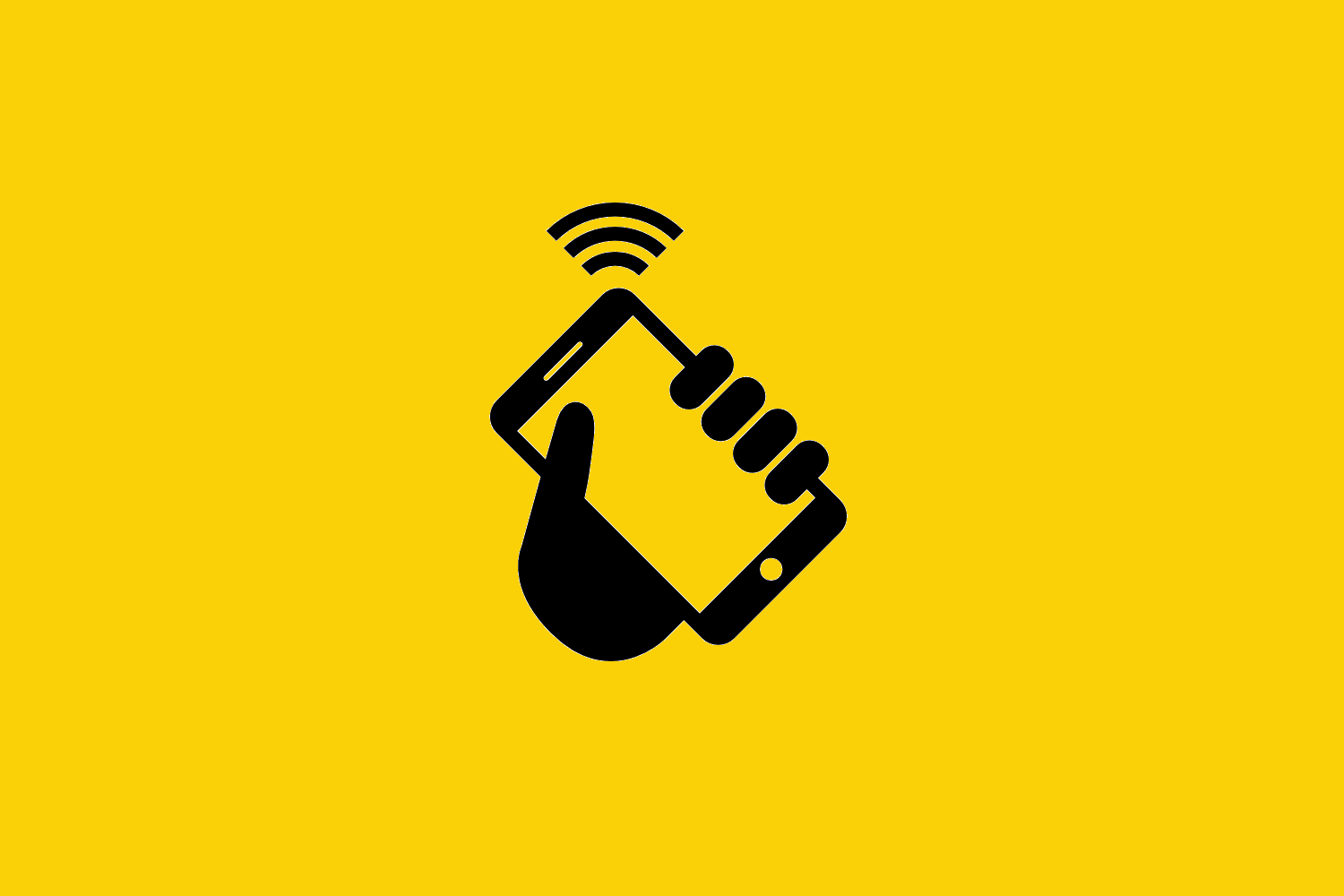
Phone Number Validation
For as long as developers have written programmes that accept user input, there has been a need for validation. Input validation may not always be the most enjoyable aspect of software development but it is key in keeping your code running as intended. (Remember, don’t trust your users.)
Phone numbers provide a means of ensuring that a record is associated with a real person and, in the case of user accounts, provide a channel through which one can recover a compromised account, as unsafe as that might sometimes be. In order to increase the chances that an accurate phone number is provided, input validation is required. Or, more specifically, phone number validation.
In a tale almost as old as time Stack Overflow, developers have sought ways to ensure that a valid phone number is provided.
Our overlords at Google saw the problem and, as they so often do, provided an elegant solution: libphonenumber
, a library “for parsing, formatting and validating international phone numbers.” It is officially available in Java, C++ and JavaScript. There are, however, ports in various languages such as Rust, Elixir and PHP. (See here for a full list.)
This article will guide you through the process of using the Python port of libphonenumbers
.
Similar functionality to what is demonstrated here should be available across the various ports of the library.
Prerequisites
- Python 3+
Installation
While not mandatory, it is highly advisable to create a virtual environment for your project.
Once done, install the package.
pip install phonenumbers
Using phonenumbers
With the package installed, you can now start using the phonenumbers
package to validate user input.
Using the package is as simple as:
>> import phonenumbers
>> parsed_number = phonenumbers.parse("+254700000000")
>> phonenumbers.is_valid_number(parsed_number)
True
For a sample implementation that reflects a potential real-world use case, see this GitHub repository which contains a simple Python script that:
- Asks a user to enter a phone number.
- Validates the phone number while handling common errors that may arise.
- Returns a message indicating whether or not the input is valid.
Other ways in which you could use the package include:
- Returning the validated phone number in various formats:
# National
>>> phonenumbers.format_number(
parsed_number, phonenumbers.PhoneNumberFormat.NATIONAL
)
'0700 000000'
# International
>>> phonenumbers.format_number(
parsed_number, phonenumbers.PhoneNumberFormat.INTERNATIONAL
)
'+254 700 000000'
# E164 (See: https://en.wikipedia.org/wiki/E.164)
>>> phonenumbers.format_number(
parsed_number, phonenumbers.PhoneNumberFormat.E164
)
'+254700000000'
- Find the phone number’s carrier.
Please note that this returns the phone number’s original carrier. If the phone number was migrated to a different carrier, the result may differ from the current value.
>> from phonenumbers import carrier
>> carrier.name_for_valid_number(parsed_number, "en")
'Safaricom'
- Find out if the country a phone_number is from supports number portability, i.e., can a number migrate from one network to another.
>> phonenumbers.is_mobile_number_portable_region("KE")
True
- Find the country a phone number is from.
>> from phonenumbers import geocoder
>> geocoder.country_name_for_number(parsed_number, "en")
'Kenya'
- Find one or more phone numbers from a block of text.
>> msg = "Please call me on 0700 000 000 in 15 minutes."
>>> for match in phonenumbers.PhoneNumberMatcher(msg, "KE"):
... print(
phonenumbers.format_number(
match.number, phonenumbers.PhoneNumberFormat.INTERNATIONAL
)
)
+254 700 000000
And there you have it, a simple solution to a problem you are likely to face if you build consumer-facing appliactions.
Should you have any questions about the snippets above or the sample application linked to on GitHub, drop us a line in the comments below.
Until next time, stay tuned.
Phone icon used in the header image created by Freepik - Flaticon